Spring Boot has 50+ libraries available to add to your application. With so much functionality, how do you know you’re not missing critical features that could avoid hours of wasted development? My answer was to spend way too long browsing official docs. But after a few years I did uncover some hidden gems my colleagues never knew existed. Here are my favourites to help you save time and eliminate unnecessary code....
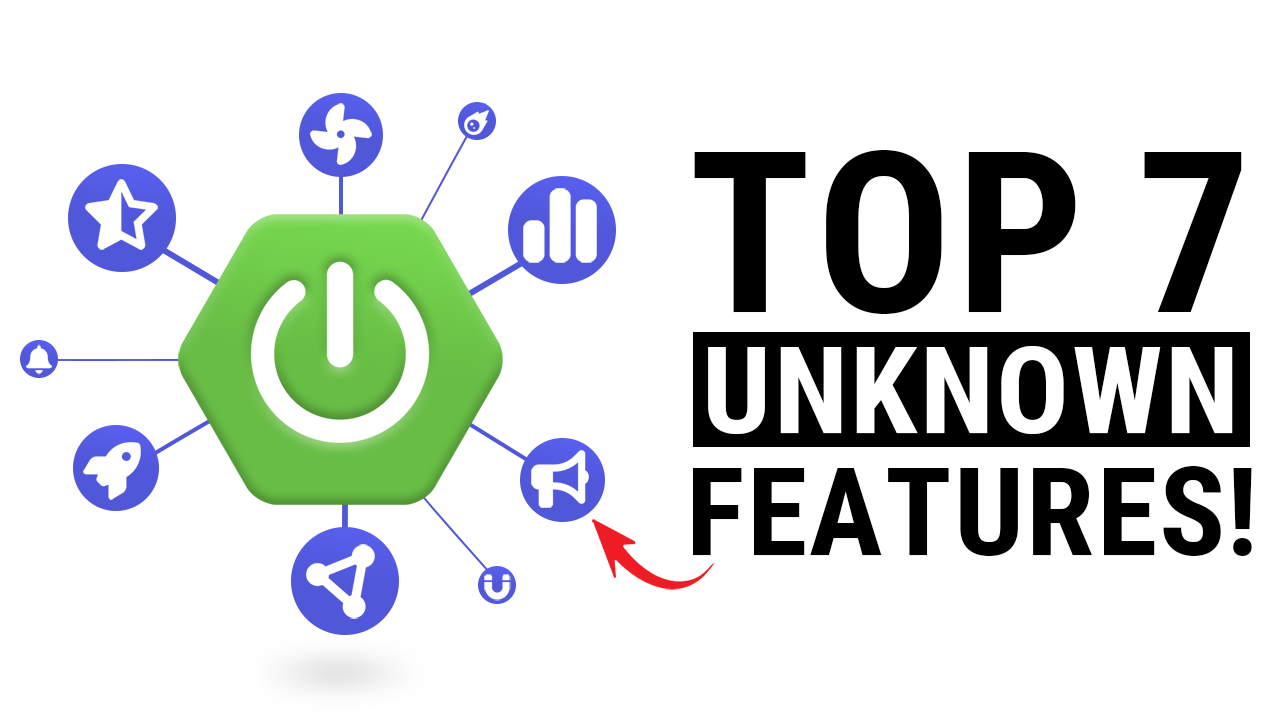